Database
Basic Setup
- Signup for an account at supabase.com or login to your existing account.
- Create a new Supabase project
- Copy your new project's Public Key, Secret Key, and URL into your codebase
AppConstants.kt
file.
Creating a new Table
- Go the the SQL editor, click the "New query" button, paste in the contents of your codebase schema.sql file and click "RUN". This will create your database tables, security policies, and triggers.
Run this query to add an example profiles table :
create table public.profiles (
id uuid not null references auth.users on delete cascade,
customer_id text,
price_id text,
has_access boolean,
email text,
primary key (id)
);
alter table public.profiles enable row level security;
- Go to the new table you created above and add 2 RLS policies:
- Enable read access for authenticated users only
- Enable insert access for authenticated users only
Here's an example Policy query to enable read access for authenticated users:
CREATE
POLICY "Grant read access to authenticated users on profile table."
ON
news_articles
FOR
SELECT
TO
authenticated_users
USING
(
TRUE
);
You can change the policies depending on how you want your app to work.
Supabase comes with a very handy AI assistant that can try down the query for you based on the prompts
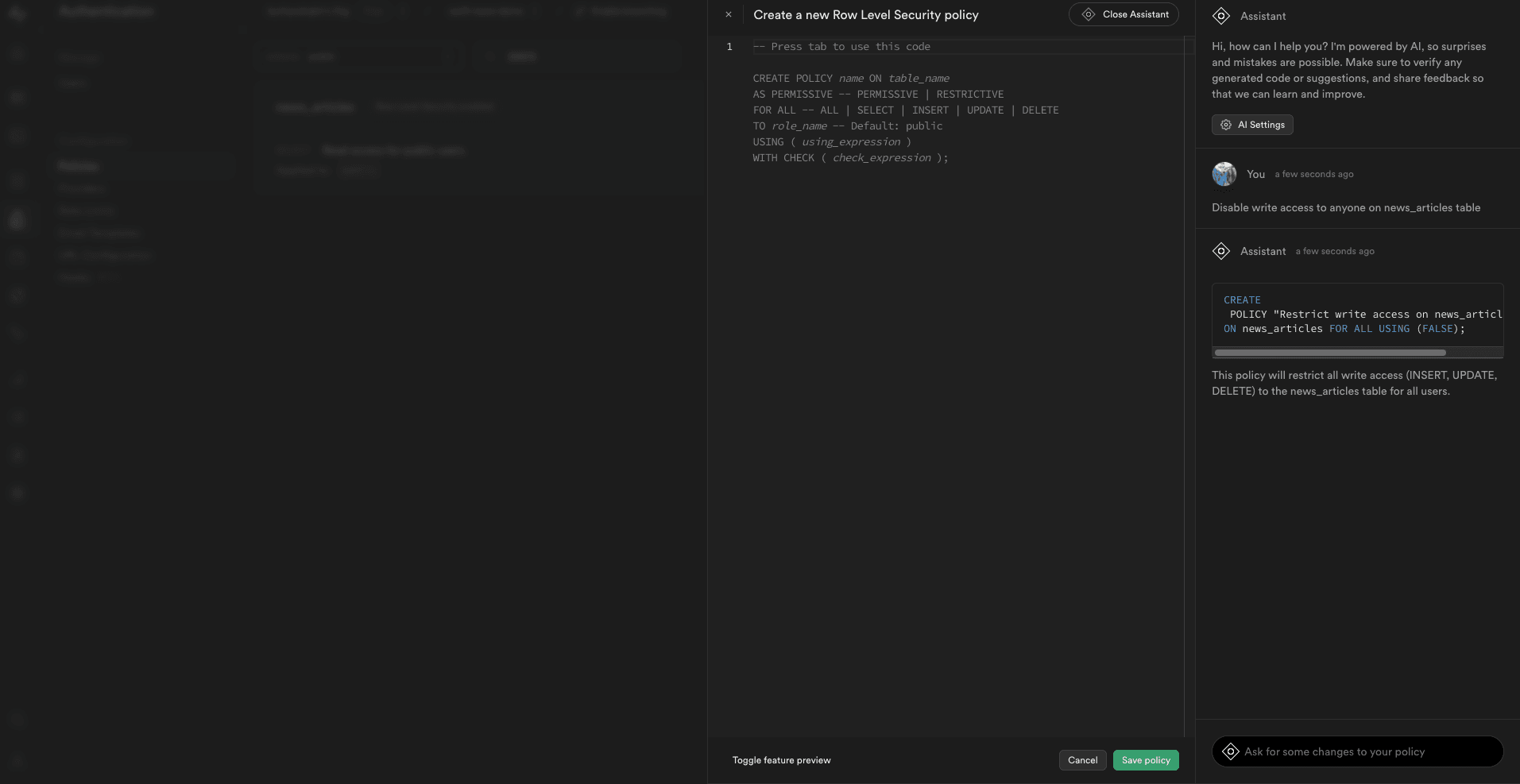
Fetching table data in the app
- Create a model class called
Profiles.kt
inside the/ui/models
directory
import kotlinx.serialization.SerialName
import kotlinx.serialization.Serializable
@Serializable
data class Profiles(
@SerialName("id")
val id: String,
@SerialName("customer_id")
val customer_id: String,
@SerialName("price_id")
val price_id: String,
@SerialName("has_access")
val hasAccess: Boolean,
@SerialName("email")
val email: String,
)
- Add the following function to the
toolbox/SupabaseRepository.kt
class
suspend fun getProfiles(): List<Feed> {
return try {
supabase.postgrest["feed"].select().decodeList<Feed>()
} catch (e: Exception) {
Timber.e("Error fetching feed items", e)
emptyList()
}
}
- Call this function from any of the Screen class like this:
val getProfiles: (() -> Unit) = {
coroutineScope.launch {
loading = true
feedList = SupabaseRepository.getProfiles()
loading = false
}
}